Debugging Happily in Yii2
Variable Dumping in Yii2 has never been so easy until you read this
In the past few days, I hear some of my friends in ‘my-ex-office’ using Yii2 as their “development framework”. In my opinion, it’s all up to developers there to decide what framework is the best for their environment and common project. But there’s a lack in Yii2 for many developers there who used to develop web app using Laravel. The dump
and dd
method. In this article, I will explain how to bring this feature to Yii2.
Using The Component
Yii2 keeps it philosophy to use component instead of Laravel Service Provider. This component is lazy load, means it will never exists on your runtime unless you need it somehow. I’m using Yii2 Component to register the dump
and dd
method.
Requiring Symfony Var Dumper Component
Laravel dump
and dd
method using Symfony Var-Dumper Component. So, we need to include this component to our composer.json
file. You can do this via command line as so:
composer require symfony/var-dumper --dev -vvv
NOTE: The
--dev
flag indicate the component is only installed in development only. Read more here.
Writing The Component
First, create a class inside components
folder right under your root application folder, and give it a name Debugger.php
:
.
├── assets
│ └── AppAsset.php
├── commands
│ └── HelloController.php
├── components
│ └── Debugger.php -> Here's your new file
[... ommited ...]
Below is the Debugger.php
content:
<?php
namespace app\components;
use Symfony\Component\VarDumper\Dumper\CliDumper;
use Symfony\Component\VarDumper\Cloner\VarCloner;
use Symfony\Component\VarDumper\Dumper\HtmlDumper;
class Debugger
{
public function dd()
{
array_map(function ($value) {
$this->dump($value);
}, func_get_args());
die(0);
}
public function dump($value)
{
if (class_exists(CliDumper::class)) {
$dumper = (PHP_SAPI === 'cli')
? new CliDumper
: new HtmlDumper;
$dumper->dump((new VarCloner)->cloneVar($value));
} else {
var_dump($value);
}
}
}
Registerring The Component
To register this component, open your configuration, find the components
section like so:
<?php
$config = [
'id' => 'basic',
'basePath' => dirname(__DIR__),
'bootstrap' => ['log'],
'components' => [
'request' => [
// !!! insert a secret key in the following (if it is empty) - this is required by cookie validation
'cookieValidationKey' => 'bUNSeF096m4EaatIrhhKSmDTPtBn6Kb8',
],
'cache' => [
'class' => 'yii\caching\FileCache',
],
// [... ommited ...]
],
'params' => $params,
];
There inside your components
configuration add this line:
'debugger' => [
'class' => 'app\components\Debugger',
],
So your final configuration like this:
<?php
$config = [
'id' => 'basic',
'basePath' => dirname(__DIR__),
'bootstrap' => ['log'],
'components' => [
'debugger' => [
'class' => 'app\components\Debugger',
],
'request' => [
// !!! insert a secret key in the following (if it is empty) - this is required by cookie validation
'cookieValidationKey' => 'bUNSeF096m4EaatIrhhKSmDTPtBn6Kb8',
],
'cache' => [
'class' => 'yii\caching\FileCache',
],
// [... ommited ...]
],
'params' => $params,
];
Using Your Debugger
You can acess your debugger anywhere with this snippet:
<?php
Yii::$app->debugger->dump('Something you want to debug here');
// Or if you want to stop PHP execution immediately after dumping variable
Yii::$app->debugger->dd('Something you want to debug here');
[BONUS] Registering Your dd
or dump
to Global Level
Sometimes you’re too lazy to type:
<?php
Yii::$app->debugger->dd('Something you want to debug here');
All you want to type is:
<?php
dd('Something you want to debug here'); // Kinda Laravel, eh?
You need to change your Debugger
class, add a constructor
and write this line:
public function __construct()
{
$this->registerMethodAliases();
}
Then add a registerMethodAliases
method inside it:
protected function registerMethodAliases()
{
require_once __DIR__.'/debugger.helpers.php';
}
You may notice that registerMethodAliases
method require a debugger.helpers.php
file inside it’s folder. So you need to make this file. Inside components
folder, create debugger.helpers.php
. To make sure, see this tree file:
.
├── assets
│ └── AppAsset.php
├── commands
│ └── HelloController.php
├── components
│ ├── debugger.helpers.php -> This is your new file
│ └── Debugger.php
And here’s debugger.helpers.php
file content:
<?php
use app\components\Debugger;
if (! function_exists('dump')) {
function dump()
{
call_user_func_array([(new Debugger()), 'dump'], func_get_args());
}
}
if (! function_exists('dd')) {
function dd()
{
call_user_func_array([(new Debugger()), 'dd'], func_get_args());
}
}
As I told before, Yii Component is lazy load, so your Debugger
class will not build unless you need it. To make this Debugger
eager load, change your config
under bootstrap
section, add debugger
in it:
<?php
$config = [
'id' => 'basic',
'basePath' => dirname(__DIR__),
'bootstrap' => ['log', 'debugger'], // Add `debugger` component here
'components' => [
'debugger' => [
'class' => 'app\components\Debugger',
],
'request' => [
// !!! insert a secret key in the following (if it is empty) - this is required by cookie validation
'cookieValidationKey' => 'bUNSeF096m4EaatIrhhKSmDTPtBn6Kb8',
],
'cache' => [
'class' => 'yii\caching\FileCache',
],
// [... ommited ...]
],
'params' => $params,
];
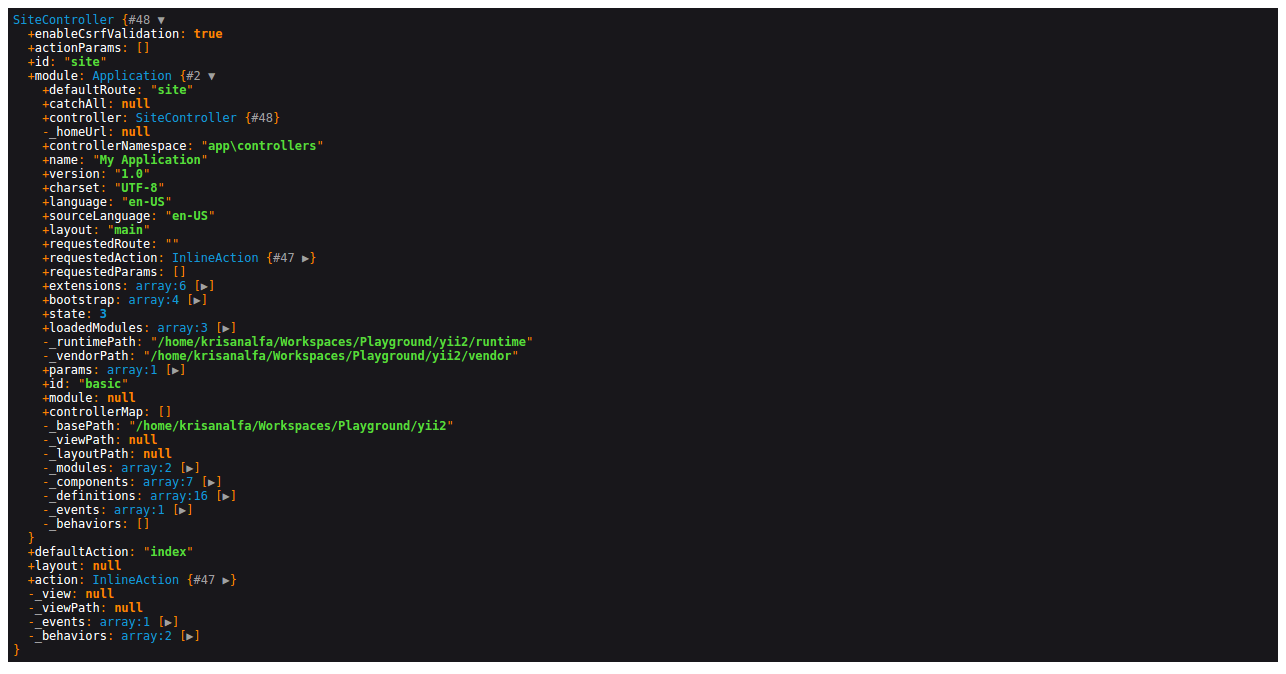
Dumping variable like a boss
After you read this tutorial, you may use dd
or dump
anywhere you want
There you go, you may access dd
or dump
function wherever you want. If you think this article is useful or maybe helpful for you, share it would be nice. Hapy code!